父模块
方式一:菜单栏 :File —> New —> Project…
方式二:启动 IDEA 后 ,选择


父模块可以直接删除 src
文件夹。
子模块 - 普通
右键父模块 —> New
—> Module...
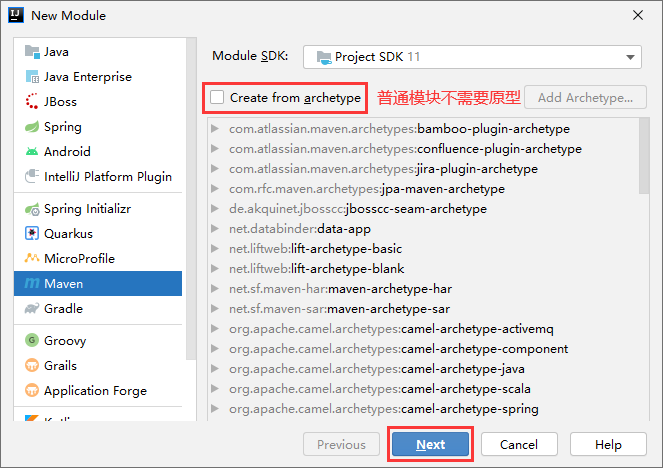
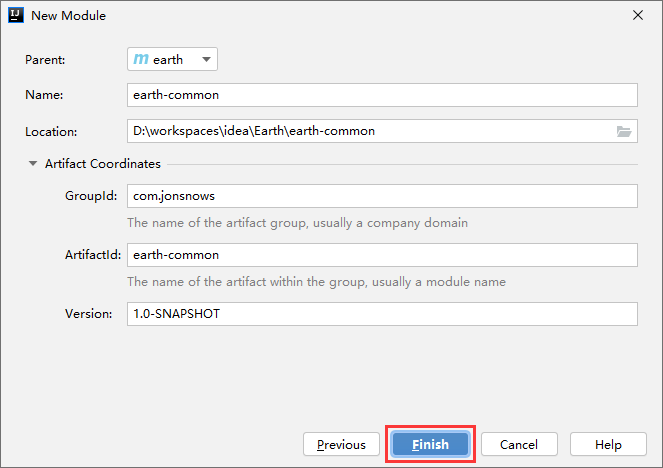
子模块 - Maven 原型 webapp
Archetype 是一个 Maven 项目模板工具包。通过 Archetype 我们可以快速搭建 Maven 项目。
常见的 Archetype:
简单的 Maven 工程原型: maven-archetype-quickstart
、 maven-archetype-simple
简单的 Maven Web 工程原型: maven-archetype-webapp
右键父模块 —> New
—> Module...
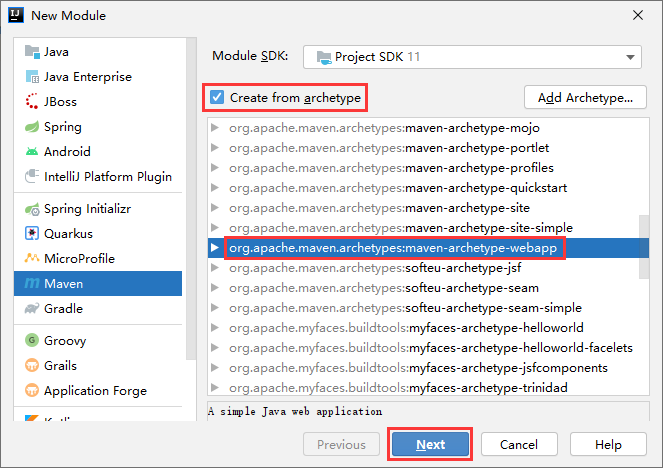
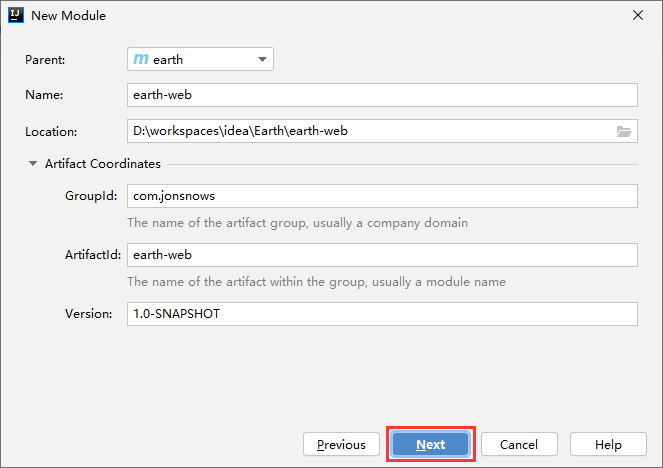

子模块 - springboot
右键父模块 —> New
—> Module...
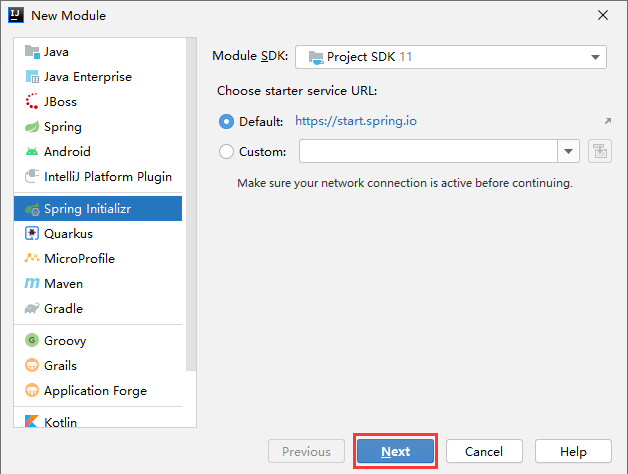
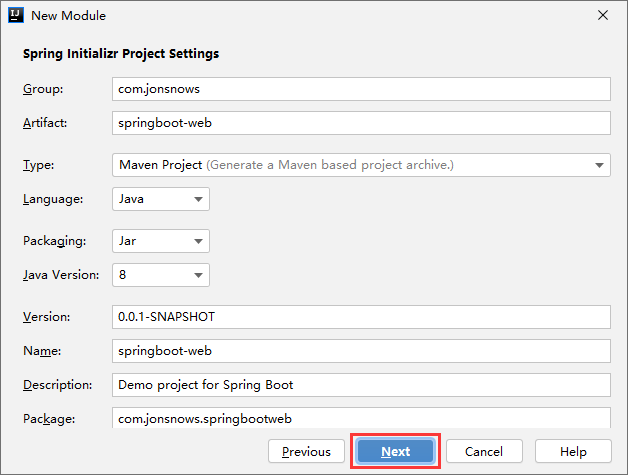
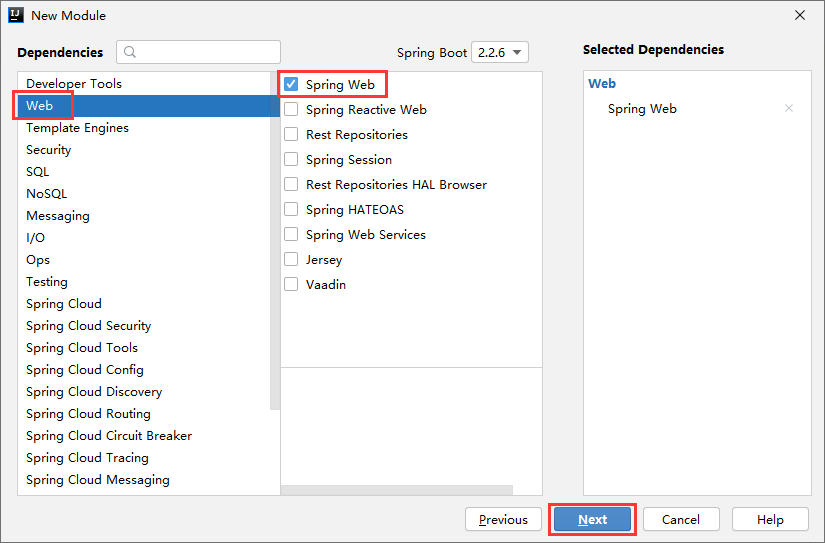
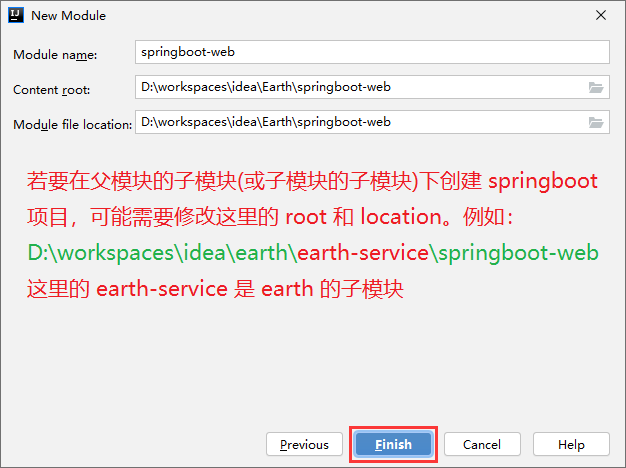
创建一个 controller 如下:
1 2 3 4 5 6 7 8 9 10 11 12
| import org.springframework.web.bind.annotation.RestController; import org.springframework.web.bind.annotation.RequestMapping;
@RestController public class HelloController {
@RequestMapping("/") public String index() { return "Greetings from Spring Boot!"; }
}
|
然后使用 spring-boot-maven-plugin
启动
微服务工程
父工程
按照上文 父模块 创建 Maven 父工程,然后在其 pom 文件引入依赖,spring Boot 版本为 2.2.6.RELEASE
,Spring Cloud 版本为 Hoxton.RELEASE
。这个 pom 文件作为父 pom 文件,起到依赖版本控制的作用,其他 module 工程继承该 pom 。代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>com.jonsnows</groupId> <artifactId>earth</artifactId> <packaging>pom</packaging> <version>1.0-SNAPSHOT</version>
<name>earth</name> <description>地球计划</description>
<modules> </modules>
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version>
<spring-boot.version>2.2.6.RELEASE</spring-boot.version> <spring-cloud.version>Hoxton.RELEASE</spring-cloud.version> <maven.plugin.version>3.8.1</maven.plugin.version> </properties>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> </dependencies>
<dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>${spring-boot.version}</version> <type>pom</type> <scope>import</scope> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
<build>
<plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <version>${spring-boot.version}</version> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>${maven.plugin.version}</version> <configuration> <source>${java.version}</source> <target>${java.version}</target> <encoding>UTF-8</encoding> </configuration> </plugin> </plugins> <pluginManagement> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <version>${spring-boot.version}</version> </plugin> </plugins> </pluginManagement> </build>
<repositories> <repository> <id>central</id> <name>Central-Repository</name> <url>https://repo.maven.apache.org/maven2/</url> <layout>default</layout> <releases> <enabled>true</enabled> </releases> <snapshots> <enabled>false</enabled> </snapshots> </repository> </repositories>
<pluginRepositories> <pluginRepository> <id>central</id> <name>Central-Repository-Plugin</name> <url>https://repo.maven.apache.org/maven2/</url> <layout>default</layout> <releases> <enabled>true</enabled> </releases> <snapshots> <enabled>false</enabled> </snapshots> </pluginRepository> </pluginRepositories>
</project>
|
服务注册中心 (Eureka Server)
右键父模块 —> New
—> Module...
,选择 spring initialir
,然后 next
:
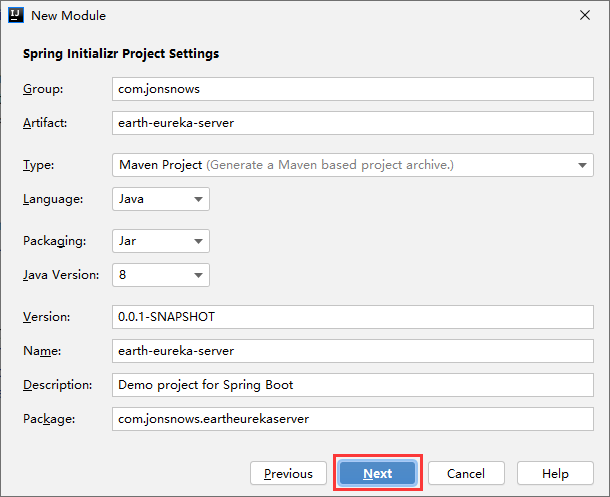
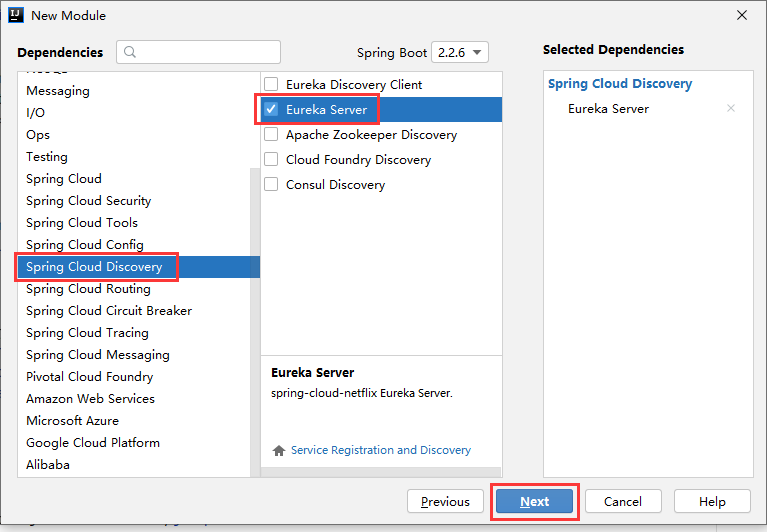
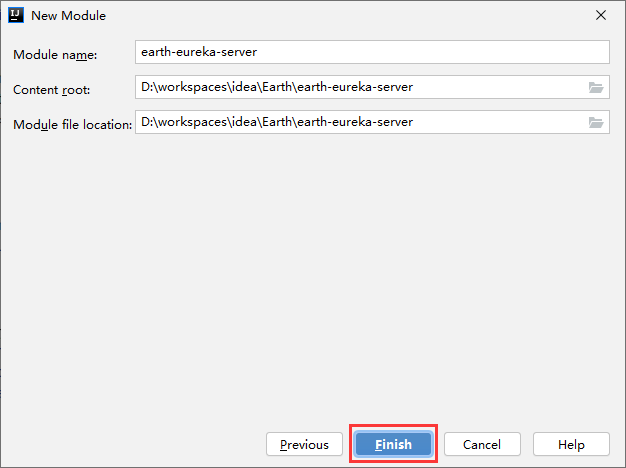
pom.xml 如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<parent> <groupId>com.jonsnows</groupId> <artifactId>earth</artifactId> <version>1.0-SNAPSHOT</version> </parent>
<groupId>com.jonsnows</groupId> <artifactId>earth-eureka-server</artifactId> <version>0.0.1-SNAPSHOT</version>
<name>earth-eureka-server</name> <description>Demo project for Spring Boot</description>
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency> </dependencies>
</project>
|
启动服务注册中心
springboot 工程的启动 application 类加上注解 @EnableEurekaServer
:
1 2 3 4 5 6 7 8
| @SpringBootApplication @EnableEurekaServer public class EarthEurekaServerApplication {
public static void main(String[] args) { SpringApplication.run(EarthEurekaServerApplication.class, args); }
|
eureka 是一个高可用的组件,它没有后端缓存,每一个实例注册之后需要向注册中心发送心跳(因此可以在内存中完成)。
在默认情况下 erureka server 也是一个 eureka client,必须要通过 eureka.client.registerWithEureka:false
和 fetchRegistry:false
来表明自己是一个 eureka server。
文件 appication.yml:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| server: port: 8761
spring: application: name: eurka-server
eureka: instance: hostname: localhost client: registerWithEureka: false fetchRegistry: false serviceUrl: defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/
|
启动工程,打开浏览器访问: http://localhost:8761 。
服务提供者 (Eureka Client)
当 client 向 server 注册时,它会提供一些元数据,例如主机和端口,URL,主页等。Eureka server 从每个 client 实例接收心跳消息。 如果心跳超时,则通常将该实例从注册 server 中删除。
创建过程同 server 类似,
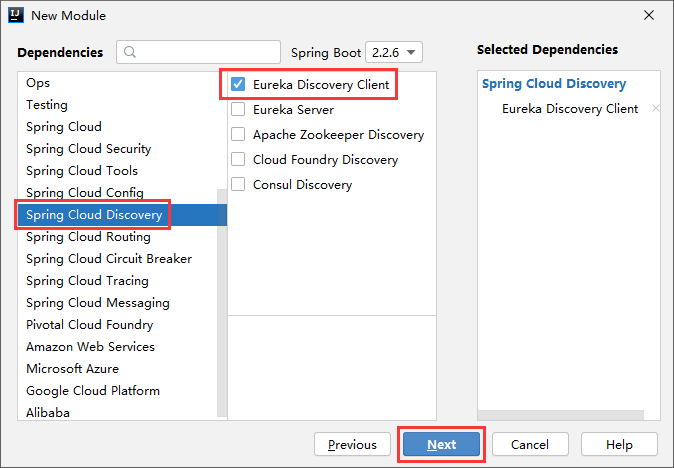
pom.xml 如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<parent> <groupId>com.jonsnows</groupId> <artifactId>earth</artifactId> <version>1.0-SNAPSHOT</version> <relativePath/> </parent>
<groupId>com.jonsnows</groupId> <artifactId>earth-eureka-client</artifactId> <version>0.0.1-SNAPSHOT</version>
<name>earth-eureka-client</name> <description>Demo project for Spring Boot</description>
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies>
</project>
|
通过注解 @EnableEurekaClient
表明自己是一个 eureka client。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @SpringBootApplication @EnableEurekaClient @RestController public class EarthEurekaClientApplication {
public static void main(String[] args) { SpringApplication.run(EarthEurekaClientApplication.class, args); }
@Value("${server.port}") String port;
@RequestMapping("/hello") public String home(@RequestParam(value = "name", defaultValue = "jonsnows") String name) { return "hello " + name + " ,i am from port:" + port; }
}
|
然后在配置文件中注明自己的服务注册中心地址,application.yml 配置文件如下:
1 2 3 4 5 6 7 8 9 10 11
| server: port: 8762
spring: application: name: eureka-client
eureka: client: serviceUrl: defaultZone: http://localhost:8761/eureka/
|
启动工程,打开 http://localhost:8762/hello?name=jonsnows
访问注册中心 http://localhost:8761/ 如下:
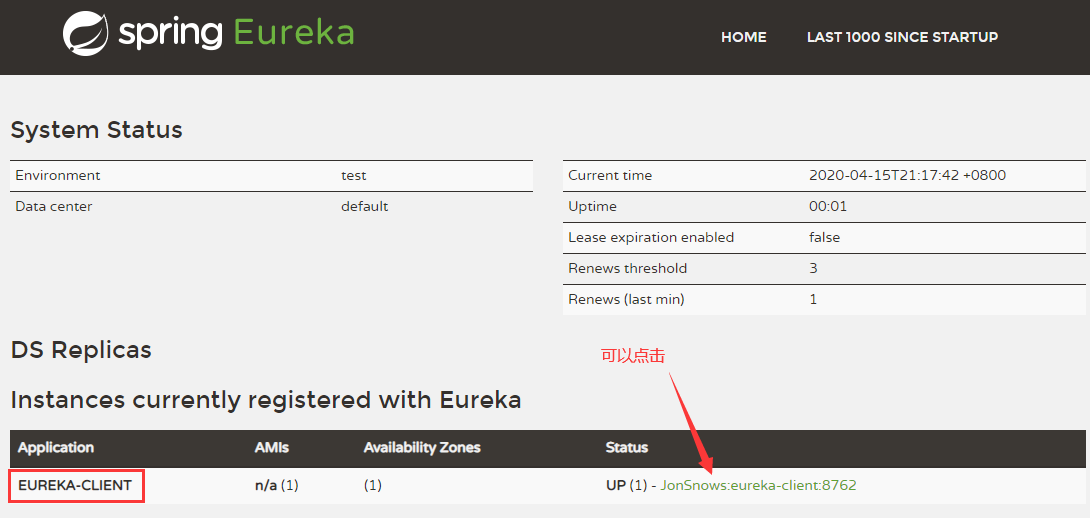